In multilevel inheritance a parent class is extended by child class and this child class is again extended by another class. Let us understand with help of a diagram:
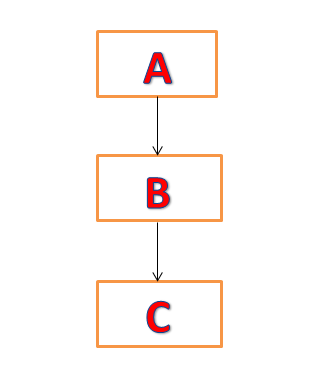
Above diagram shows an example of multilevel inheritance. Class B extends class A and class C extends class B.
- Here the members of class A will be accessible by the object of class C, although class C is not directly extending class A.
- Also the members of class A can be directly used inside class C.
Let us look at the java code to understand the relationship:
public class Tech4Humans {
public static void main(String[] args) {
C obj = new C();
//object of class C is able to access members of class A due to multilevel inheritance
obj.var1=20;
obj.var2=30;
obj.methodA();
obj.methodC();
System.out.println("var1="+obj.var1);
System.out.println("var2="+obj.var2);
}
}
class A{
int var1;
void methodA() {
System.out.println("method in class A");
}
}
class B extends A{
int var2;
void methodB() {
System.out.println("method in class B");
}
}
class C extends B{
int var3;
void methodC() {
System.out.println("method in class C");
//members of class A are directly accessible inside class C due to multilevel inheritance
System.out.println("printing variable of class A = "+var1);
System.out.println("printing variable of class B = "+var2);
}
}
Output
method in class A
method in class C
printing variable of class A = 20
printing variable of class B = 30
var1=20
var2=30
Let us take a look at another type of inheritance – Hierarchical inheritance.