In Hierarchical inheritance a class is inherited by more than one classes. Let us take a look at the diagram:
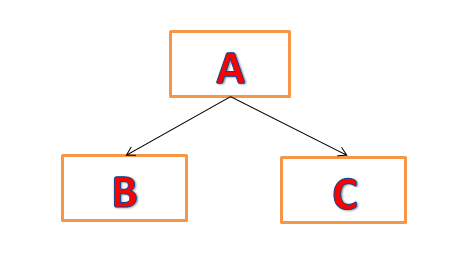
In the above diagram we can see that class B and class C is inheriting from the same class A which is called Hierarchical Inheritance in Java.
Let us look at an example to understand more clearly:
public class Tech4Humans { public static void main(String[] args) { Son objSon = new Son(); Daughter1 objDaughter = new Daughter1(); //Objects of class Son and Daughter1 are able to access class Father variables objSon.skinColor="Brown"; objDaughter.skinColor="Brown"; //object of class Son and Daughter1 is able to access Father class properties objSon.fatherMethod(); objDaughter.daughterMethod(); } } class Father{ String skinColor; void fatherMethod() { System.out.println("Inside Father method"); } } class Son extends Father{ String education; void sonMethod() { System.out.println("Inside son method"); } } class Daughter1 extends Father{ String education; void daughterMethod() { System.out.println("Inside daughter method"); //We can access members of Father class directly in Daughter1 System.out.println("Skin color is "+skinColor); } }
Output
Inside Father method
Inside daughter method
Skin color is Brown
The above code shows and example of Hierarchical inheritance where classes Son and Daughter1 are inheriting Father class.
Let us take a look at Multiple Inheritance.