This is most basic type of inheritance. In single level inheritance the child class is derived from its parent class only.
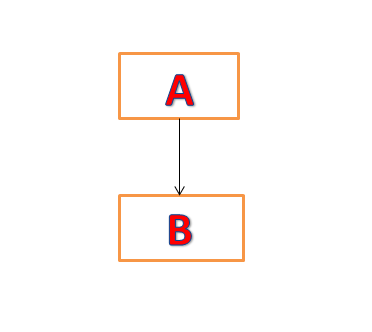
We can see from above image that class B is being derived from class A. This scenario is called single level inheritance. Let us understand with help of Java code:
public class Tech4Humans {
public static void main(String[] args) {
Daughter daughter1 = new Daughter();
daughter1.eyeColor = "Blue";
daughter1.education = "Engineer";
daughter1.getEyeColor();
daughter1.getEducation();
}
}
class Mother {
String eyeColor;
void getEyeColor() {
System.out.println("Eye color is " + eyeColor);
}
}
//Single Level Inheritance
class Daughter extends Mother {
String education;
void getEducation() {
System.out.println("Education qualification is " + education);
}
}
Output
Eye color is Blue
Education qualification is Engineer
In above code Daughter class is derived from Mother class, hence it is single level inheritance.
If Daughter class would also have been inherited by another class then it would have become multilevel inheritance. Let us look at multilevel inheritance.