In selection sorting each element is compared with all the following elements of the array. If the element is greater than any following element then swapping is done.
After the first cycle we obtain the smallest element at the starting. Now in the second cycle, second element is compared with all following elements to obtain the second smallest element and the cycle continues until we find reach till end.
Let us look at the code and then its explanation to understand more clearly:
public class Tech4Humans {
public static void main(String[] args) {
Integer[] arr = { 50, 90, 10, 5 };
linearSorting(arr);
}
static void linearSorting(Integer[] arr) {
System.out.print("Before sorting = ");
for (int k = 0; k < arr.length; k++) {
System.out.print(arr[k] + " ");
}
// Linear Sorting starts here
int temp, size = arr.length;
for (int i = 0; i < size - 1; i++) {
for (int j = i + 1; j < size; j++) {
// swapping condition
if (arr[i] > arr[j]) {
temp = arr[j];
arr[j] = arr[i];
arr[i] = temp;
}
}
}
System.out.print("\nAfter Sorting = ");
for (int k = 0; k < arr.length; k++) {
System.out.print(arr[k] + " ");
}
}
}
Output
Before sorting = 50 90 10 5
After Sorting = 5 10 50 90
Explanation
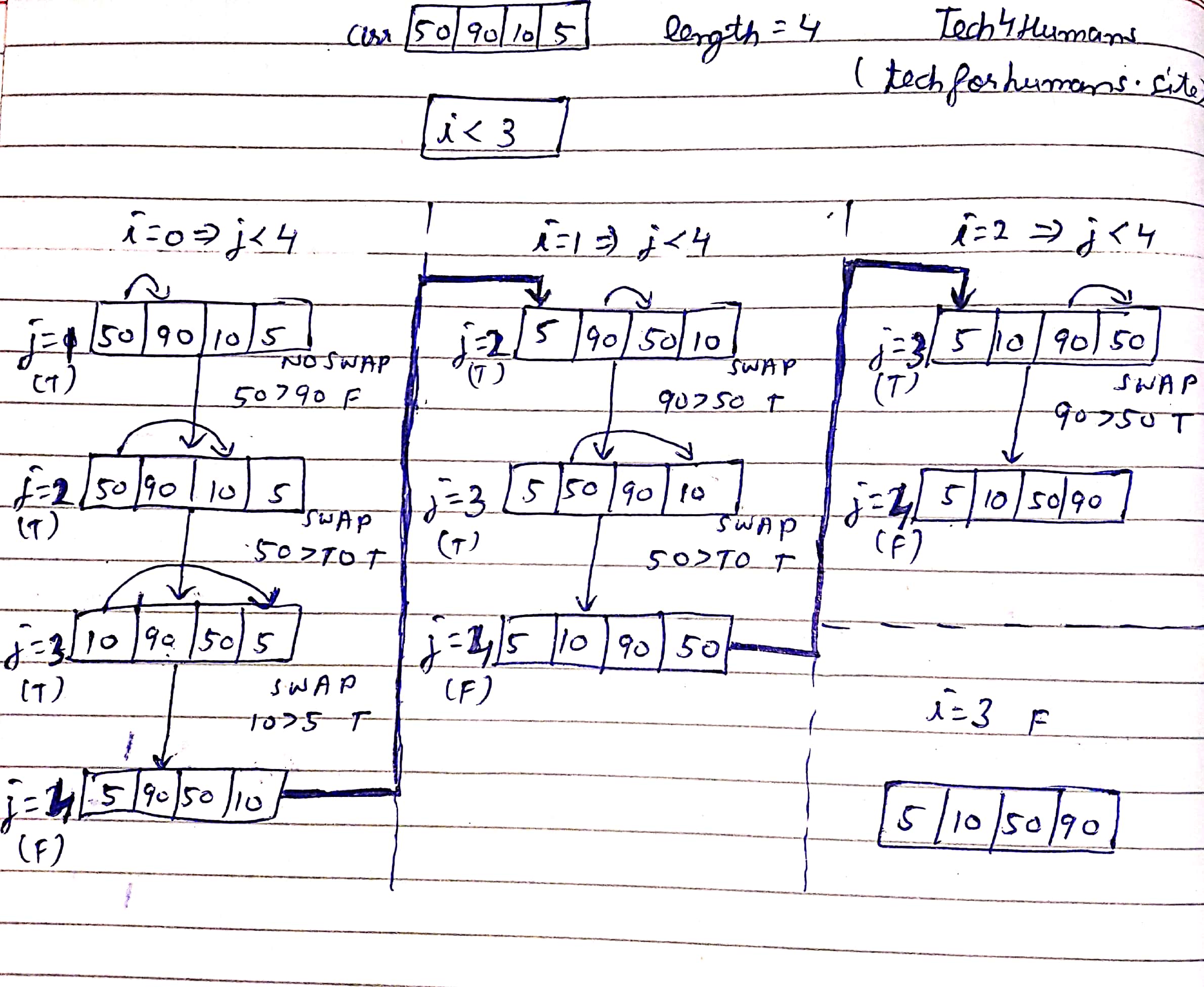
Please note in the above example that i=0, i=1…. is considered cycle first, cycle second respectively. Whereas j=0, j=1, j=2… is used to handle the comparison and swapping of elements.
Observe in first cycle(i=0), that first element is being compared to every following element and if it is greater then swapping is done. After the first cycle we obtained the smallest element(5) as the first element. Now in second cycle we compare second element of array with all the following elements, by this we get second smallest element at second position of array. This cycle goes on until we reach till end.
Complexity
Complexity of selection sorting is О(n2).
Now let us look at an optimized sorting algorithm – Quicksort.