What is Debugging?
Debugging of the code helps us to understand execution of the program line by line. In debugging we can see what is value of variables after each line which can help us understand logic of code and identify errors or bugs.
Debugging can be done manually using pen and paper or by using tools such as Eclipse.
How to debug in Eclipse step by step:
Step 1 : Create a new program with class name ‘Tech4HumansDebug’ and write given below code :
public class Tech4HumansDebug {
static int sumMethod1(int num1, int num2) {
int sum = 0;
sum = num1 + num2;
return sum;
}
static int sumMethod2(int a, int b) {
int sum = 0;
for (int i = 0; i < b; i++) {
a = a + 1;
}
sum = a;
return sum;
}
public static void main(String[] args) {
int x1 = 2;
int y1 = 3;
int sum1 = sumMethod1(x1, y1);
int sum2 = sumMethod2(5, 4);
System.out.println("sum from method1 = " + sum1);
System.out.println("sum from method2 = " + sum2);
}
}
Step 2 : We have to choose a place in code where we need to start debugging our code and insert a breakpoint. A breakpoint is the line of code from where we need to debug our code and proceed manually.
To insert a breakpoint right click at the left hand side of program where line numbers are written and click on ‘Toggle Breakpoint’ as shown in below image. A breakpoint will be inserted. Alternatively we can also double click on the line number to insert breakpoint.
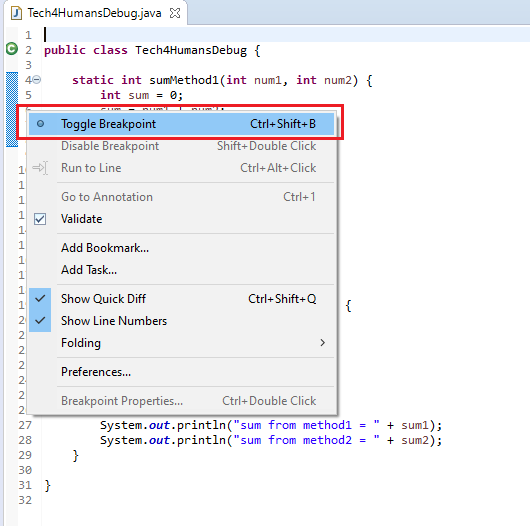
Step 3 : Similarly insert breakpoint at line 21 of our code too. There should be 2 circles(breakpoints) inserted at line 6 and 21 as shown below.

Step 4 : Now to start debugging the code right click on the code and select ‘Debug as’ and then ‘Java Application’.
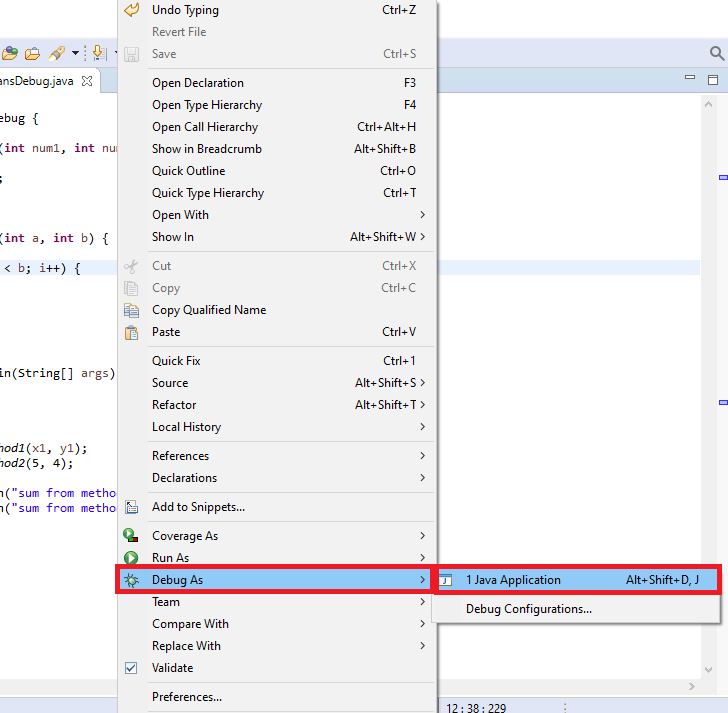
Step 5 : A new dialog box will appear which will ask to change perspective. Click on ‘Switch’ button.
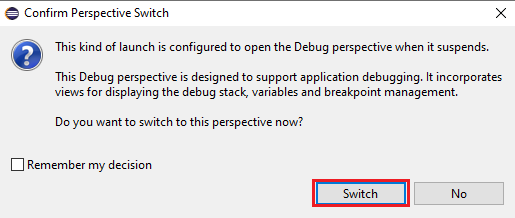
Step 6 : By changing perspective some additional windows will appear on eclipse which will help you debug code easily. The statement with first breakpoint will be highlighted with a green debug pointer. On the tool bar few buttons will be enabled too which were previously disabled. Let us understand one by one.
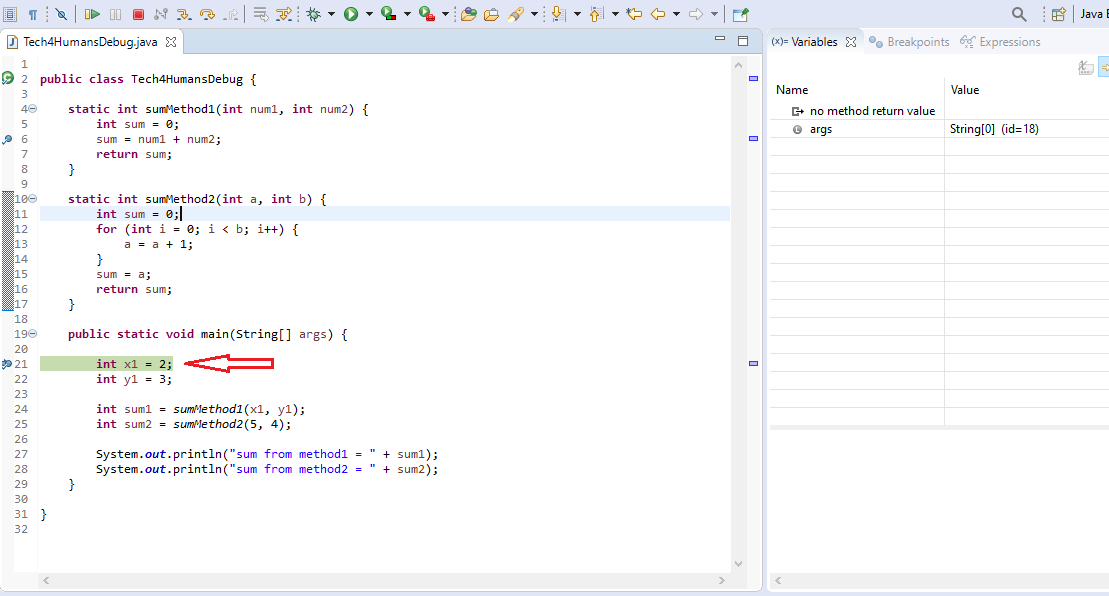
At the right hand side a new window will appear having 3 tabs ‘Variables’, ‘Breakpoints’, ‘Expressions’.
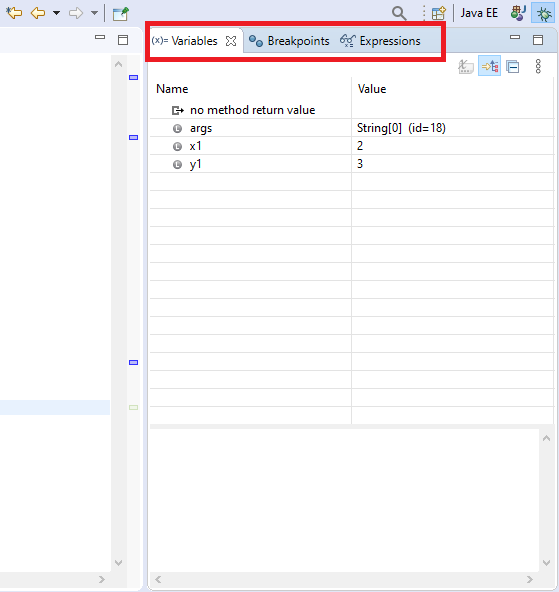
- Variables tab :- This tab shows the current value of the variables in the program. As you progress in the code the change in values of variables is reflected here.
- Breakpoints tab :- This tab shows the list of breakpoints inserted in program.
- Expressions tab :- While debugging if we want to execute any expression based on the current values of variables then we use Expressions tab.
Now let us understand the buttons in the tool bar that are enabled :-
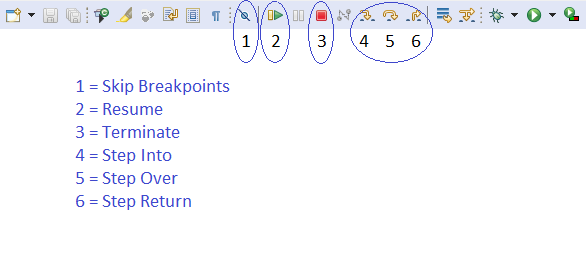
- Step Over :- On reaching a breakpoint we need to proceed line by line so that we can analyze changes in code and variables. For this we press Step over button.
- Step Into :- While proceeding step by step using ‘Step Over’ if we encounter any function call and press again Step Over on function call then instead of going inside the function the debug pointer will execute function and proceed to next statement after function call. So to get inside the function we press Step Into on reaching the function call.
- Step Return :- After reaching inside function if we want to return from body of function to function call then we press Step Return.
- Skip all Breakpoints :- Use this button if you want to skip all the breakpoints and continue with normal execution of program.
- Resume :- When we want to move from one breakpoint to next breakpoint without debugging the code in between them.
- Terminate :- If we want to exit debugging and stop program execution.
Step 7 : Now press on the Step Over button in the tool bar and you will observe the value of variables being updated in the variables tab.
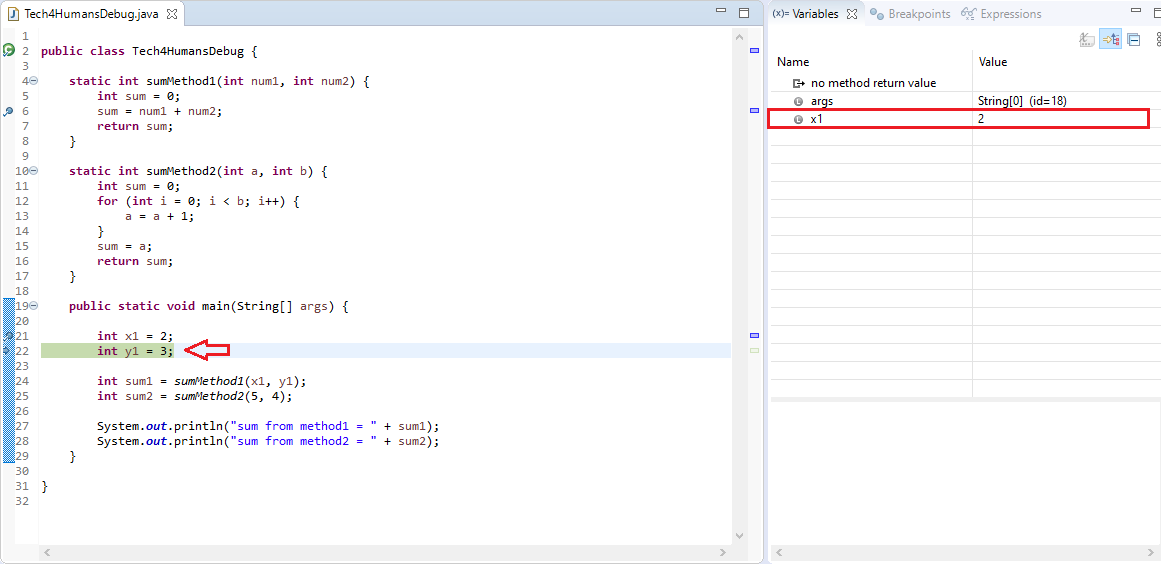
Step 8 : Similarly press Step Over once more to reach the function call.
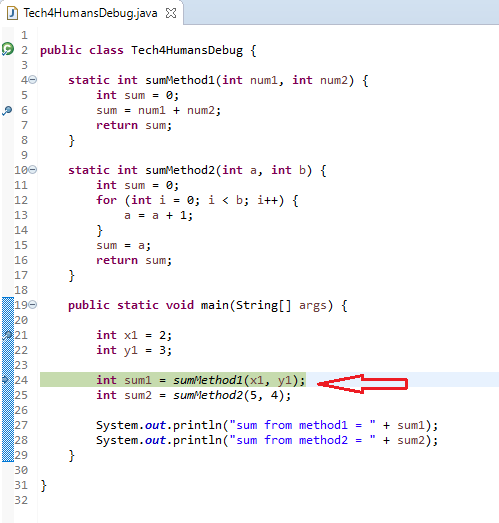
Step 9 : Now press Step Into to get inside the function body. The variables inside the function body can also be observed in the variables tab.
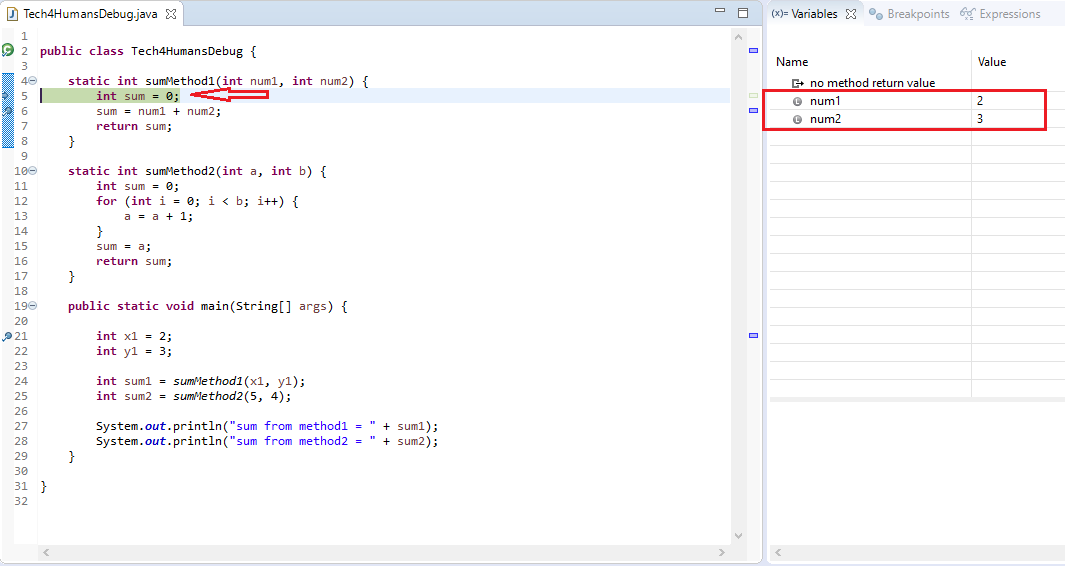
Step 10 : Now press step over to move to next statement.
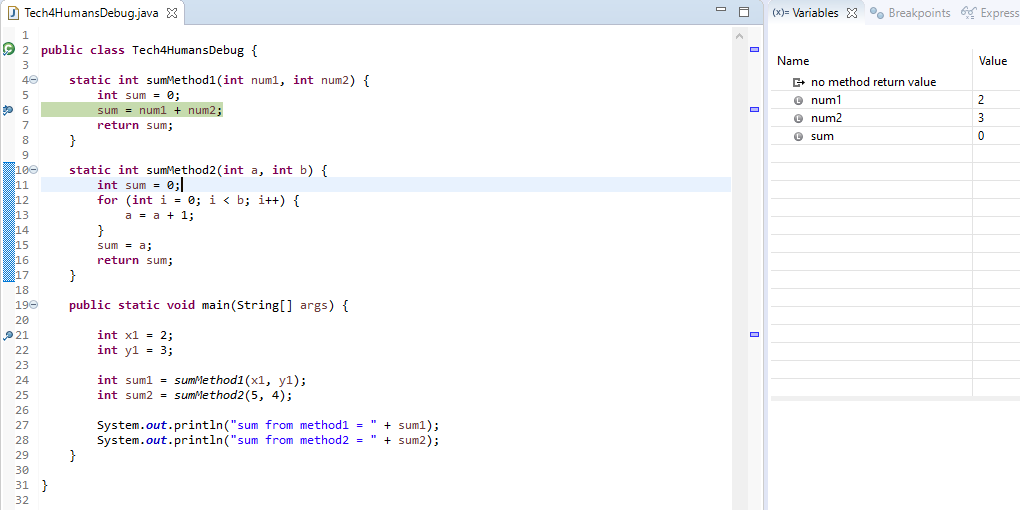
Step 11 : Now you can proceed in a similar way i.e. press Step Over to move to last statement of function and press Step Over again to return to function call(Step Return can also be used to return). Now on reaching to 25th line again press Step Into to enter inside the function sumMethod2 and so on.
Let us see how to Import code in Eclipse.